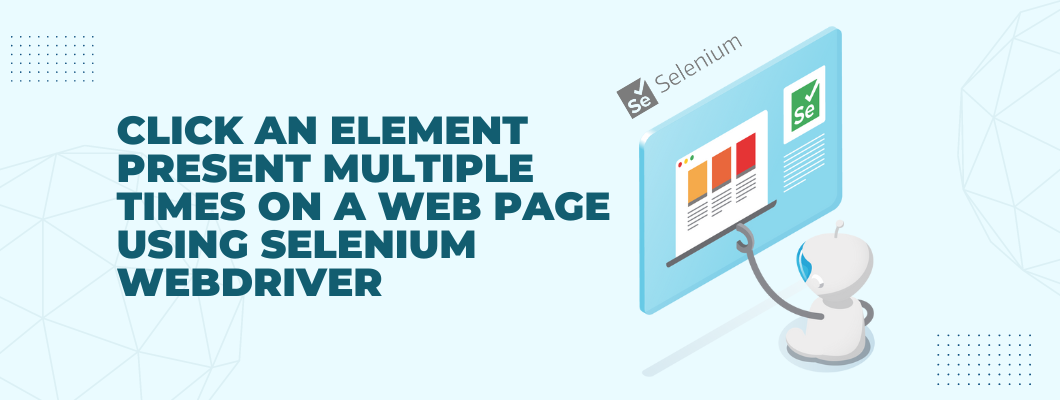
How to Select Multiple Wishlist buttons In Selenium?
When there are multiple options and multiple elements provided for the same, we can code it in such a way that on executing the code all the elements would be selected. So, let us look at the code for handling multiple elements.
There are different ways in Selenium using which we can select the elements on a Web Page. We are selecting the element using Xpath.
Let’s take an example as Flipkart. When we click on the Like button and add that product to the Wishlist. But now we want to select all the products in the particular category.
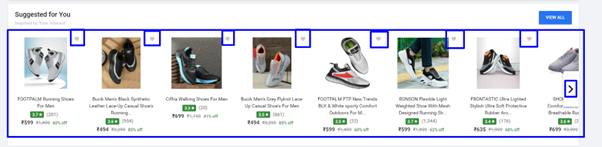
For that, we can add the following conditions and loop to selecting all the Like buttons.
//Write the condition for one particular category for selecting the Like button.
if (driver.findElement(By.xpath("(//*[@class='_37K3-p'])[3]")).isDisplayed()) {
//Calculate the similar element for selecting category
List<WebElement> heartButton = driver.findElements(By.xpath("//*[@class='_36FSn5']"));
System.out.println("Number of Wishlist : " + Integer.toString(heartButton.size()));
for (int index = 0; index < heartButton.size(); index++) {
//Below condition is for scrolling righthand side
if (index != 0 && index % 6 == 0 && driver.findElement(By.xpath("(//*[@class='_35l9rN _31Mq1b'])[3]")).isDisplayed()) {
WebElement leftScrolling = wait.until(ExpectedConditions.elementToBeClickable(By.xpath("(//*[@class='_35l9rN _31Mq1b'])[3]")));
leftScrolling.click();
}
WebElement selectButtons = heartButton.get(index);
selectButtons.click();
Thread.sleep(2000L);
}
}
Thus, we can handle multiple same elements. This was just an example, and you can try it with any other website where multiple same elements are present.
Thus, multiple same elements can be handled with the help of Selenium.
Full code file Flipkart.java
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.interactions.Actions;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.openqa.selenium.JavascriptExecutor;
import org.testng.annotations.Test;
import java.io.IOException;
import java.time.Duration;
import java.util.List;
public class Flipkart {
@Test(groups = {"Flipkart"})
public void Flipkartwhishlist() throws IOException, InterruptedException {
System.setProperty("webdriver.chrome.driver", "C:\\browserdriver\\chromedriver.exe");
ChromeOptions options = new ChromeOptions();
options.addArguments("--window-size=1920,1080");
WebDriver driver = new ChromeDriver(options);
Actions act = new Actions(driver);
driver.manage().window().maximize();
driver.manage().deleteAllCookies();
//Visit App URL
driver.get("https://www.flipkart.com/");
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(20));
//Enter email ID
WebElement enterEmail = wait.until(ExpectedConditions.elementToBeClickable(By.xpath("(//input[@type='text'])[2]")));
enterEmail.sendKeys("XYZ@gmail.com");
//Enter Password
WebElement SubmitPass = wait.until(ExpectedConditions.elementToBeClickable(By.xpath("//input[@type='password']")));
SubmitPass.sendKeys("Password");
//Click on Sign In button
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.xpath("(//button[@type='submit'])[2]")));
element.click();
Thread.sleep(5000L);
// Maximize browser
driver.manage().window().maximize();
// Create instance of Javascript executor
JavascriptExecutor je = (JavascriptExecutor) driver;
//Identify the WebElement which will appear after scrolling down
WebElement firstScrolling = wait.until(ExpectedConditions.elementToBeClickable(By.xpath("//h2[contains(text(),'Beauty, Food, Toys & more')]")));
// now execute query which actually will scroll until that element is not appeared on page.
je.executeScript("arguments[0].scrollIntoView(true);", firstScrolling);
//Identify the WebElement which will appear after scrolling down
WebElement SecondScrolling = wait.until(ExpectedConditions.elementToBeClickable(By.xpath("(//*[@class='Nyt1vx'])[1]")));
// now execute query which actually will scroll until that element is not appeared on page.
je.executeScript("arguments[0].scrollIntoView(true);", SecondScrolling);
//Write condition for one particular category for selecting Like button.
if (driver.findElement(By.xpath("(//*[@class='_37K3-p'])[3]")).isDisplayed()) {
//Calculate the similar element for selecting category
List<WebElement> heartButton = driver.findElements(By.xpath("//*[@class='_36FSn5']"));
System.out.println("Number of Wishlist : " + Integer.toString(heartButton.size()));
for (int index = 0; index < heartButton.size(); index++) {
//Below condition is for scrolling righthand side
if (index != 0 && index % 6 == 0 && driver.findElement(By.xpath("(//*[@class='_35l9rN _31Mq1b'])[3]")).isDisplayed()) {
WebElement leftScrolling = wait.until(ExpectedConditions.elementToBeClickable(By.xpath("(//*[@class='_35l9rN _31Mq1b'])[3]")));
leftScrolling.click();
}
WebElement selectButtons = heartButton.get(index);
selectButtons.click();
Thread.sleep(2000L);
}
}
System.out.println("All WishList have been added");
}
}
Result
For Run, the code right-click and click the highlighted menu item.
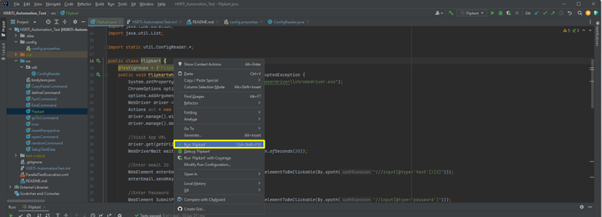
After the execution of the code, the final result is following:
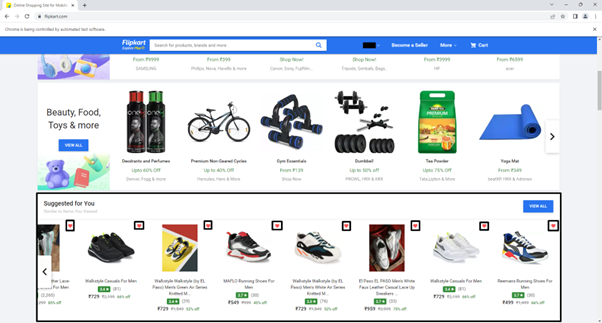
Terminal:-
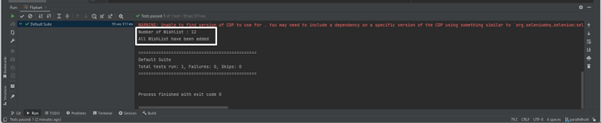